How To Delete Thousands of Tweets on Twitter/X
If you've been using Twitter/X for a long time, you likely have accumulated thousands of posts. Deleting them to clear up your online presence may become necessary if your personal circumstances change. Fortunately, deleting tweets using Python is easy.
Requirements
- Python 3
- Archive of Twitter Data (Free! Just Req
jq
installed- Twitter/X API permissions (Free Tier OK!)
Getting Started
First, get a Twitter/X API key. Go to the Twitter Developer Portal and login if you're not already.
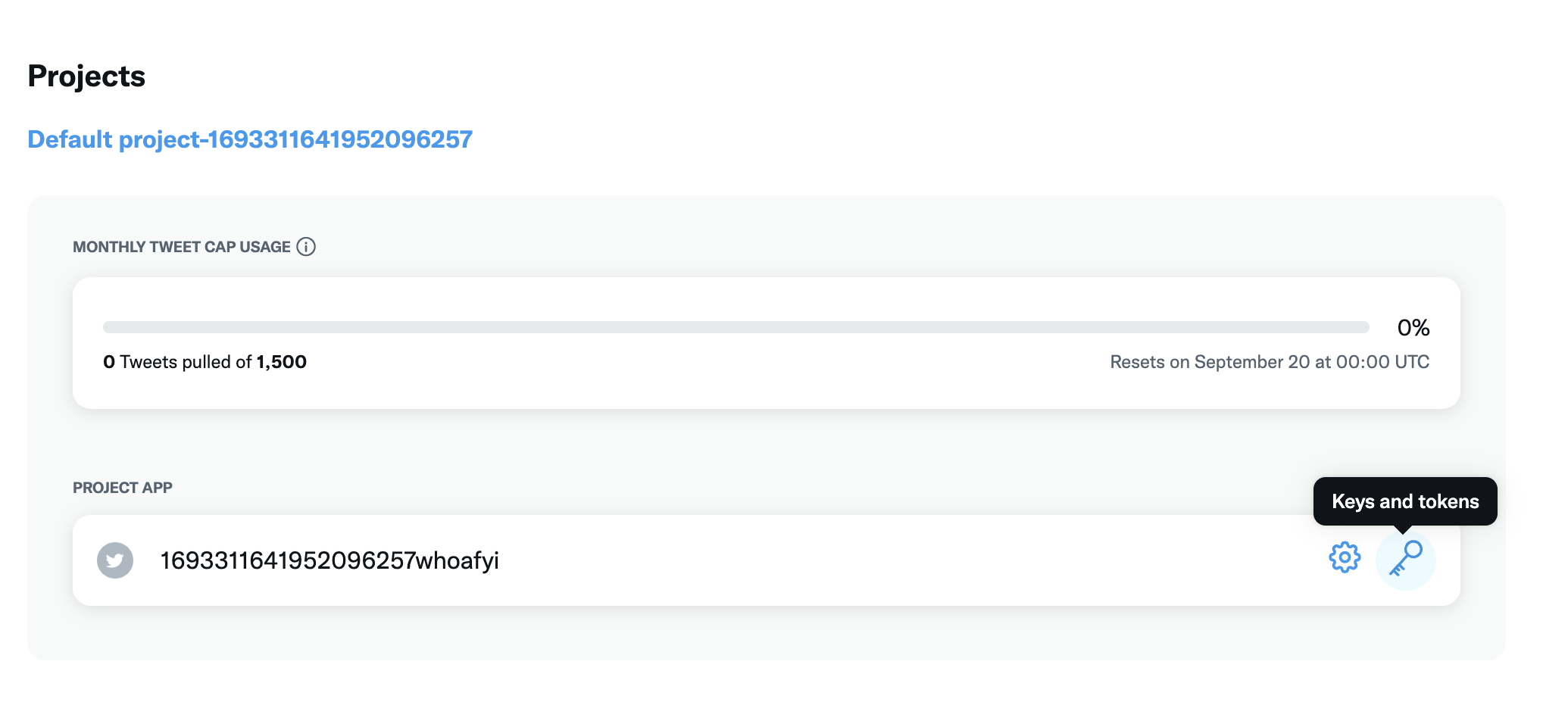
On the dashboard, you should have a default project already created.
Click on Keys and Tokens.
Generate an API Key and Secret under 'Consumer Keys', save both of them in a file.
Generate an Access Token and Secret, under 'Authentication Tokens', and save both of those in a file as well.
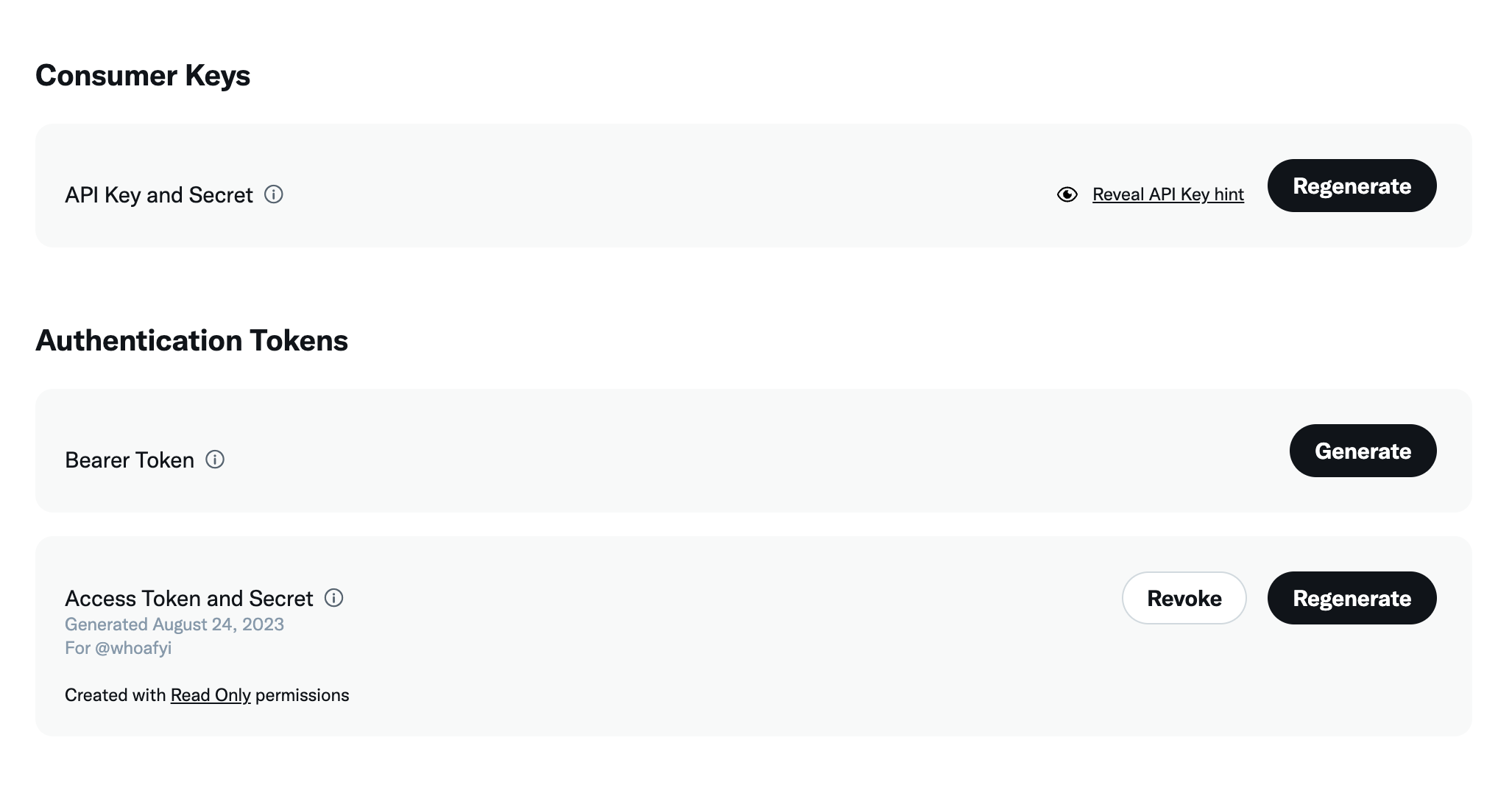
Obtain an Archive of Your Twitter Data
In order to get the list of tweet IDs, you have a few options. With the API you can get a list of your tweets, but you'll have to pay for the search feature.
Alternatively, you can download an archive of your Twitter data, which doesn't require you to pay anything and has some additional benefits.
Requesting the archive takes seconds but the actual file takes some time to generate. You can request the archive from your Twitter settings page.
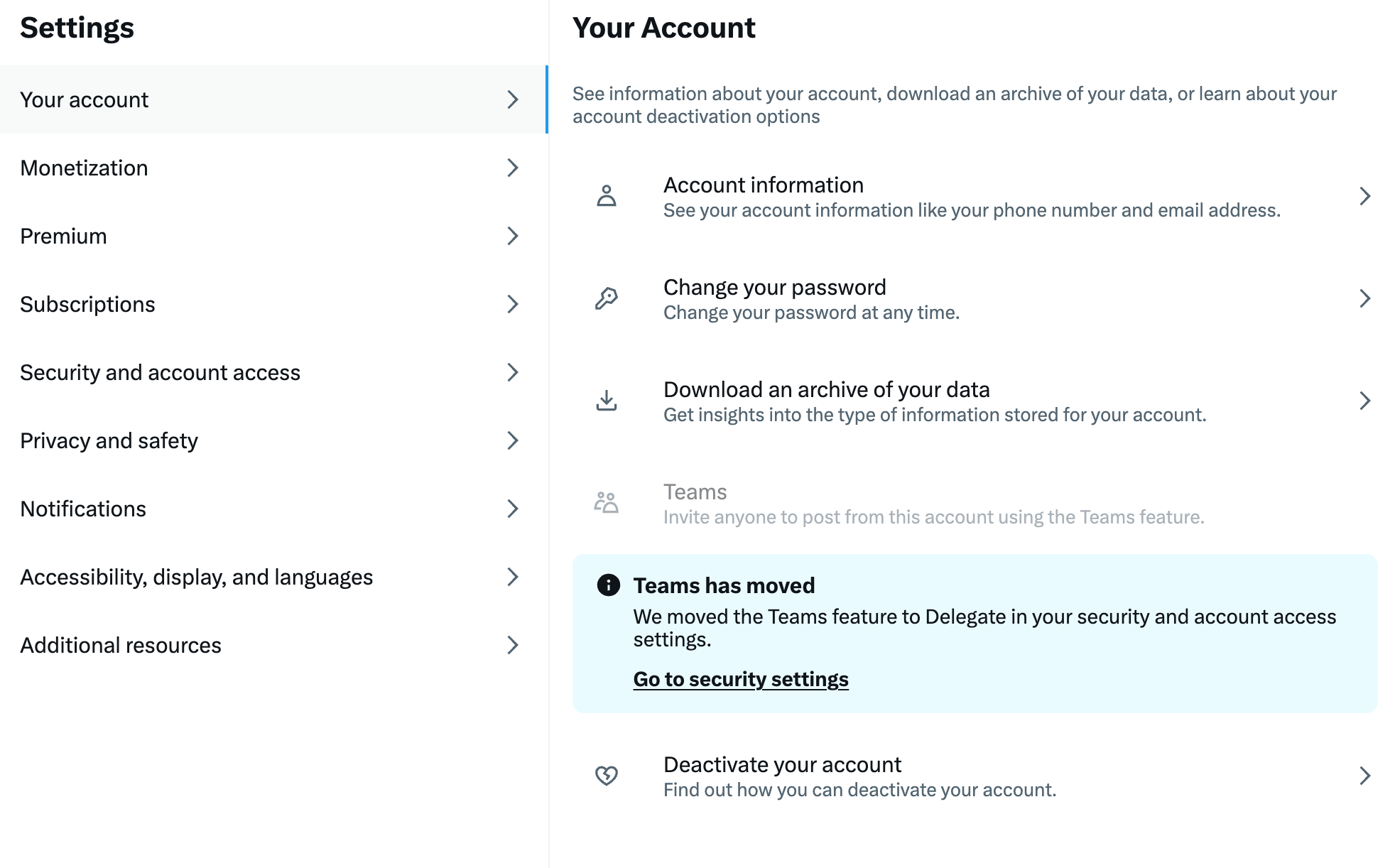
You'll get a notification when the archive is ready to download. It can be multiple gigabytes in size, depending on how much you've used Twitter.
Once the archive is ready, download it and extract it to a folder. If you wish, you can open the 'Your archive.html'
file to get a fun local webpage that lets you browse and search for data in the archive.
Obtain a List of Tweet IDs
Within the archive there is a data/tweets.js
file. This file contains an enormous JSON blob that has all of your tweets.
Make a copy of the file.
cp data/tweets.js data/tweets_copy.js
Remove the window.YTD.tweets.part0 =
characters from the top of the copied file. The objective is to have the file just be one big JavaScript array.
[
{
"tweet" : {
"edit_info" : {
"initial" : {
"editTweetIds" : [
...
Use jq
to get a list of Tweet IDs
If you're like me, you may just want to remove years of tweets in one go. You can use Google or ChatGPT to get a jq
query that will work for you.
I used this one to select all tweets created before May 1st, 2023. You can selectively search for Tweets with certain words, or to certain people, or whatever you like. Run the command in your preferred terminal.
cat data/tweets_copy.js | jq '.[] | select(.created_at < "Thu May 01 00:00:00 +0000 2023") | .tweet.id' > delete_these.txt
This will produce a list of Tweets in the delete_these.txt
file. We'll use this file as input for our Python script.
Use Python and tweepy to delete your tweets
Create a new Python virtual environment. Or don't, I'm not your dad.
Install tweepy
.
pip install tweepy
Create a new Python file, saving the contents below. Remember to update the credentials!
import tweepy
# Define your Twitter API credentials
CONSUMER_KEY = 'CONSUMER_KEY_YOU_SAVED'
CONSUMER_SECRET = 'CONSUMER_SECRET_YOU_SAVED'
ACCESS_TOKEN = 'ACCESS_TOKEN_YOU_SAVED'
ACCESS_TOKEN_SECRET = 'ACCESS_TOKEN_SECRET_YOU_SAVED'
# Set up Tweepy authentication
auth = tweepy.OAuthHandler(CONSUMER_KEY, CONSUMER_SECRET)
auth.set_access_token(ACCESS_TOKEN, ACCESS_TOKEN_SECRET)
api = tweepy.API(auth)
# Load list of tweet IDs from a file
# File needs to be in same directory or provide the correct relative path (obviously)
with open("delete_these.txt", "r") as file:
tweet_ids = file.read().splitlines()
# Loop through each tweet ID and attempt to delete the tweet
count = 0
for tweet_id in tweet_ids:
try:
try:
tweet_id = tweet_id.replace("\"", '')
api.destroy_status(tweet_id)
count += 1
print(f"Successfully deleted tweet with ID: {tweet_id}")
except tweepy.TweepyException as e:
print(f"Error deleting tweet with ID: {tweet_id}. Reason: {e}")
except Exception as e:
print(f"Error deleting tweet with ID: {tweet_id}. Reason: {e}")
print(f"Successfully deleted {count} tweets!")
print("Done!")
Run the program.
python delete_tweets.py
It will take some time for the program to run. As of writing this article, it doesn't appear that there's any real rate-limiting for deleting your tweets. It took a few hours for the script to work through about 15k messages.
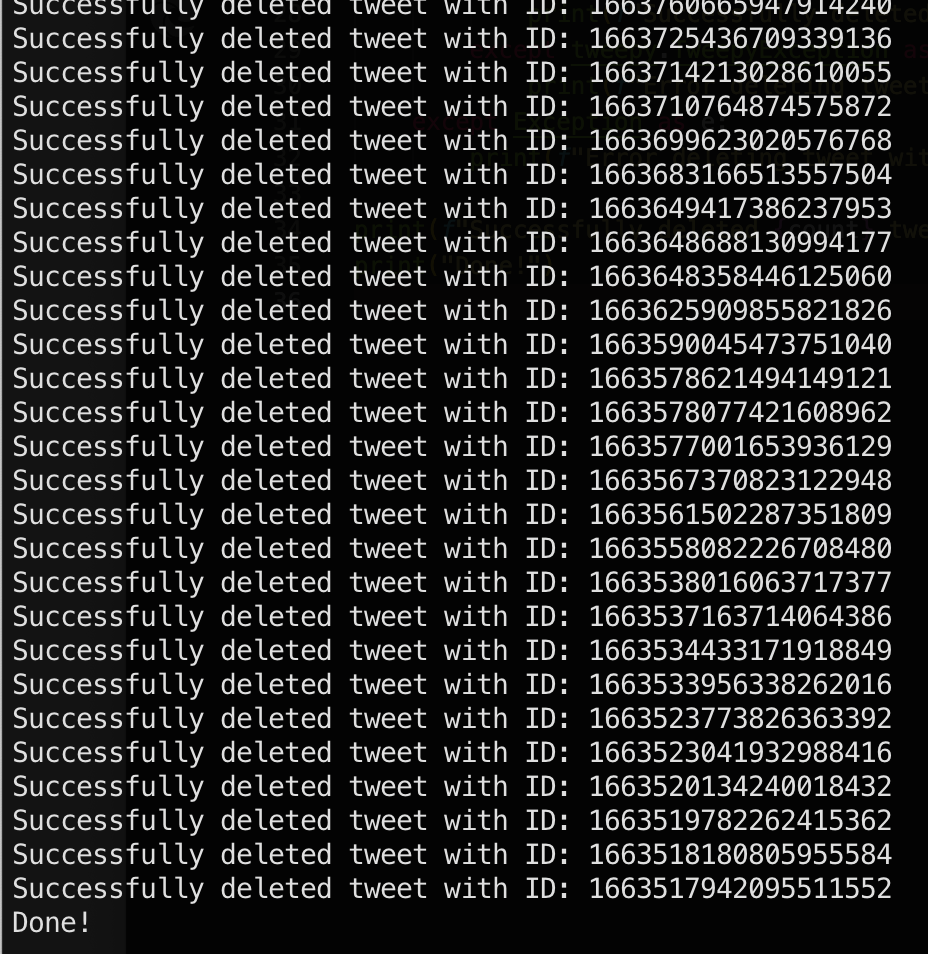
You can set up a workflow that does this periodically with cron. It's not necessary to pay a service to do this.
Conclusion
Deleting huge numbers of tweets is easy to do. You may like keeping your account as an online diary, but circumstances in your life may cause you to want to reduce personal information persisted online.