How To Get Better at LeetCode
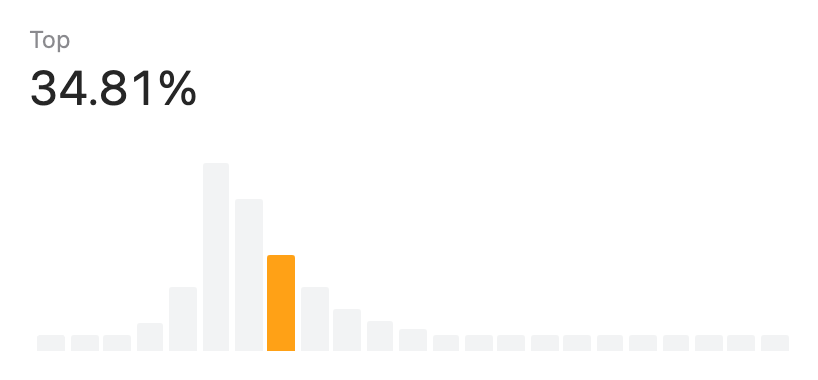
I've been doing LeetCode for the better part of six months now. During this time I've gained a bit of insight on how to improve at solving these types of algorithmic problems, which is just about necessary if you want to get into a higher-tiered technology job right now. Here are some of my observations.
Buy LeetCode Premium (or not)
LeetCode is a for-profit site that makes money from selling access to editorials and other content. Paying for a premium subscription unlocks the editorials as well as some other benefits (weekly questions for +35 leetcoins, debugger, some other stuff). During Black Friday they typically offer a sale where the subscription is 50% off, but if you think about the delta in income from getting a better job, it pays for the subscription immediately. It's less than $200 a year, so for me it's worth it.
Understand the Fundamentals
Before jumping into problems, spend some time on LeetCode Explore and get a grasp of the fundamentals. Virtually all LeetCode problems require an application of some data structures and/or algorithms, and you'll waste your time if you don't have an understanding of these basics before jumping in.
Focus on understanding different data structures (like arrays, linked lists, trees, graphs, stacks, and queues) and algorithms (such as sorting, searching, recursion, dynamic programming, and greedy algorithms). Understanding these fundamentals will make tackling problems much easier.
Keep in mind that certain problems may be particularly hard to grasp (dynamic programming is still one for me), but give yourself time to work through it.
Practice Approach
Going through some explore problems is a good way to get started. Once you finish some of those problems, you should get started on some "Easy" difficulty problems. I find it helpful to work off of a popular list of problems, found at Grind75.
The list of problems at Grind75 is a popular one - it starts out with easy problems, progressing into medium and hard difficulty problems as the weeks pass on. You can extend your practice schedule and it'll show additional problems for you to work on (currently 169). This list is a good one because it captures many of the popular problem variants you can expect to see in interviews.
There are a few different approaches to solving problems. One is to sit at a problem, work at it, try a lot of different approaches, and only after quite some time go look at the answer. I find this to be a bad approach. Instead, spend 20 minutes working on a problem, if you get stuck, then go look at the Editorial or comments section for a solution. Read that solution carefully, understand what it's doing, type it in or adjust your solution to accommodate it, submit the problem and move on.
The important thing about practicing is doing enough problems that you start to recognize the patterns of solutions and can implement some code motifs, e.g "I need to use BFS here..."
from collections import deque
queue = deque()
queue.append(first_node)
while queue:
node = queue.popleft()
# process stuff
Grind Problems
Show up every day and grind a problem if you're not working out of a list. If you're actively interviewing, knocking out a list and spending an hour or two every day doing problems will get you through the list pretty quickly. Depending on how smart you are (I'm not), you'll start to see improvement within months.
If you're stuck on a problem and want to get another explanation (since the editorial explanations often are not written in plain English), you can try watching NeetCode videos for a high-quality explanation. He typically puts up the solution to the daily problem within a few hours of it being posted, and his solution is usually optimal or at least very easy to implement in an interview setting.
Be Patient With Yourself
In my opinion, LeetCode problems test for analytical/problem solving thinking in a narrow space. Although the problems can be worded in a variety of creative ways, they all come down to doing some manipulation or trick that is obvious to the clever and not so obvious to the normal. I'm not particularly clever, so I rely on pattern recognition and repetition to approximate the thought process of a much-cleverer person.
There are some problem that only the gigaminds would recognize from the get-go, e.g Max Points On A Line, wherein the optimal solution requires one to notice you should the 2-argument arctangent! So obvious!
You're not likely to run into these problems unless interviewing for top-tier companies and unlucky. I find the fact these problems do get asked particularly amusing - it's selecting for people who are very, very intelligent BUT ALSO of low agency that they want to have a job working in some megacorp. Interviewers - just ask mediums, it's fine. You're not impressing anyone.
My Results
I got a job, it was fine. In all of the onsites I've done in the last year since starting my "LeetCode hobby", the coding round has been easy. Notably, I've not targeted Tier 1 companies, but this has been more than enough.
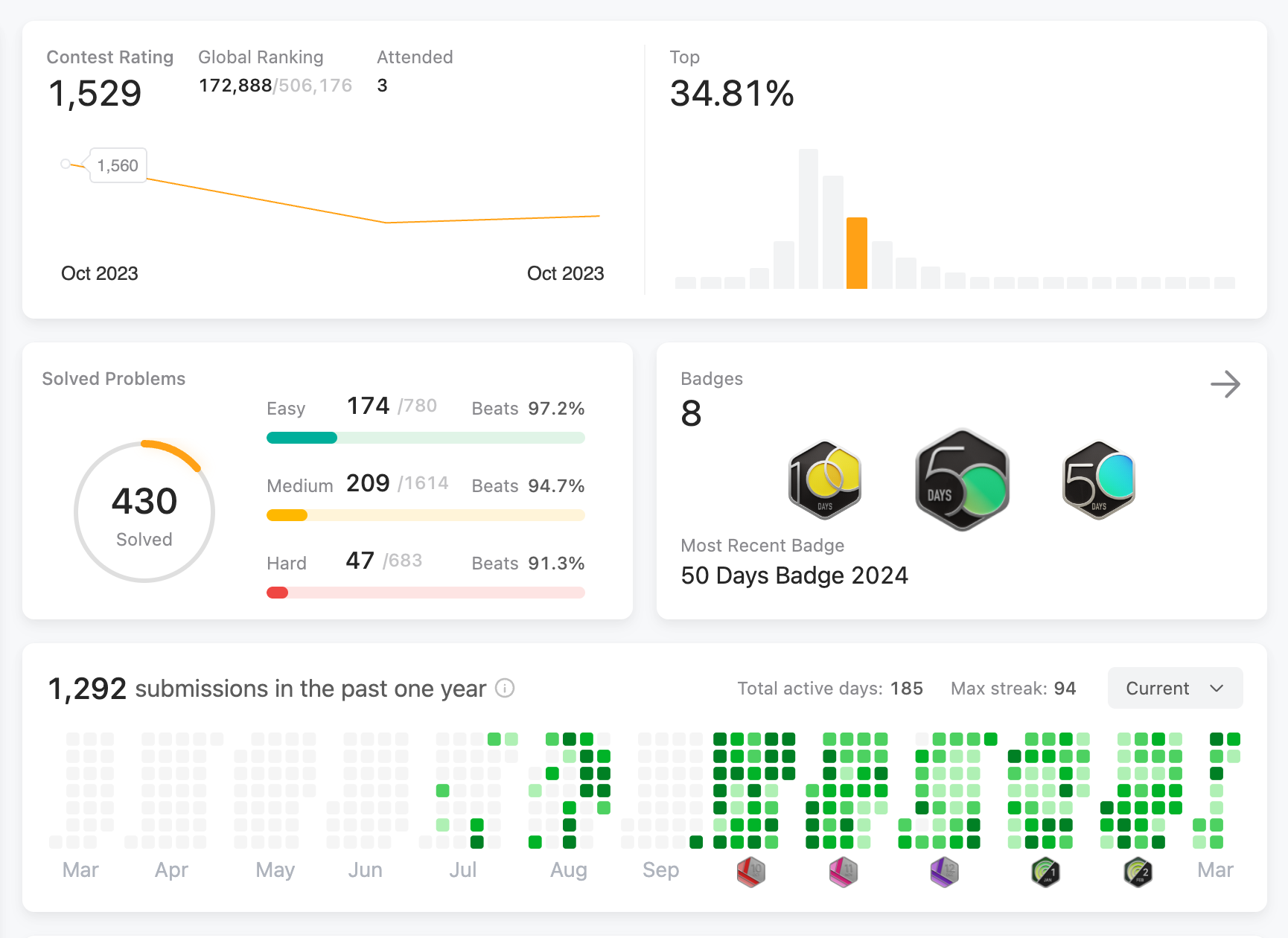
One downside: I am now addicted to leetcode. I actually look forward to the problem rolling over in the evening. I get disappointed when it's another easy problem. I hunger for leetcode. leetcode is love, leetcode is life.